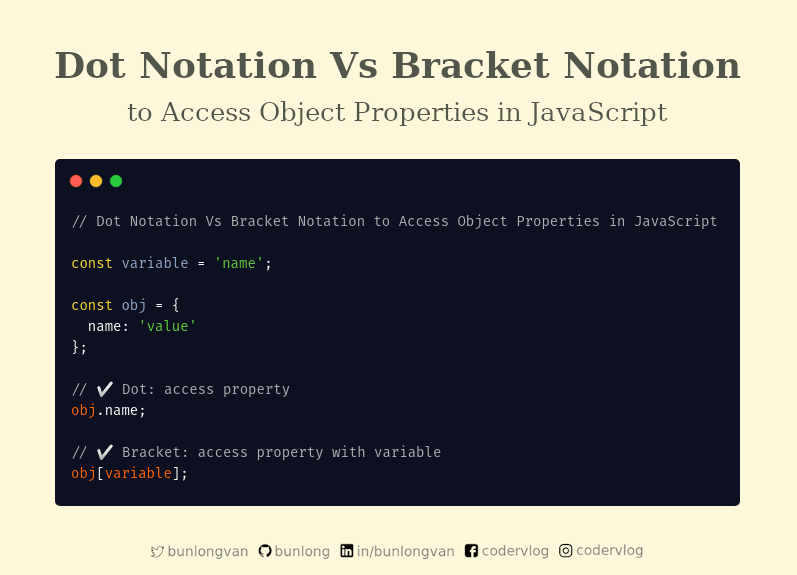
Dot Notation Vs Bracket Notation to Access Object Properties in JavaScript
Accessing Object Properties
There are 2 ways to access object properties are Dot and Bracket in JavaScript.
const obj = {
name: 'value'
};
// Dot Notation
obj.name; // 'value'
// Bracket Notation
obj['name']; // 'value'
But the question is always which one should I use 🤔. Let’s get to understand Dot Notation’s issues which is the root to answer the question.
Dot Notation’s Issues
- Issue working with invalid Identifiers
- Issue working with Variables
1. Issue working with invalid Identifiers
Dot notations is only work with valid identifiers. But what is identifiers?
Identifiers are names. In JavaScript, identifiers are used to name variables (and keywords, and functions, and labels).
In JavaScript, the identifier has the following rules:
- case sensitive
- can contain Unicode letters
- $, _, and digits (0–9) are allowed but may not start with a digit
Let’s take a look the examples below and see what happens when we use the Dot notation and Bracket notation.
const obj = {
123: 'digit',
123name: 'start with digit',
name123: 'does not start with digit',
$name: '$ sign',
name-123: 'hyphen',
NAME: 'upper case',
name: 'lower case'
};
Using with Dot Notation.
obj.123; // ❌ SyntaxError
obj.123name; // ❌ SyntaxError
obj.name123; // âś… 'does not start with digit'
obj.$name; // âś… '$ sign'
obj.name-123; // ❌ SyntaxError
obj.'name-123';// ❌ SyntaxError
obj.NAME; // âś… 'upper case'
obj.name; // âś… 'lower case'
Using with Bracket Notation.
obj['123']; // âś… 'digit'
obj['123name']; // âś… 'start with digit'
obj['name123']; // âś… 'does not start with digit'
obj['$name']; // âś… '$ sign'
obj['name-123']; // âś… 'does not start with digit'
obj['NAME']; // âś… 'upper case'
obj['name']; // âś… 'lower case'
If you think you have an invalid JavaScript identifier as your property key, use the Bracket Notation.
2. Issue working with Variables
Another issue of the Dot notation is working with variables.
Let’s take a look the examples below and see what happens when we use the Dot notation and Bracket notation.
const variable = 'name';
const obj = {
name: 'value'
};
// Dot Notation
obj.variable; // undefined
// Bracket Notation
obj[variable]; // âś… 'value'
Never use the Dot Notation when using a Variable.
Undefined Property (Bonus)
When you try to access a property that doesn’t exist, it will return undefined.
const emptyObj = {};
emptyObj.name; // undefined
emptyObj['name']; // undefined
Summary
Use the Dot Notation. Anyway if you’re dealing with invalid identifier or variables, use the Bracket Notation instead.
Thanks for reading ❤
Say Hello! Twitter | Github | LinkedIn | Facebook | Instagram